One of the essential features of java programming is Object because java is pure object oriented programming. Classes are blueprint or prototype of an object. Here's I want to show you what is Java class and object example.
Object is the physical as well as logical entity where as class is the only logical entity
Object in Java
Object is an instance of a class. Class is a template or blueprint from which objects are created. So object is the instance(result) of a class.
state: represents data (value) of an object.
behavior: represents the behavior (functionality) of an object such as deposit, withdraw etc.
identity: Object identity is typically implemented via a unique ID. The value of the ID is not visible to the external user. But, it is used internally by the JVM to identify each object uniquely.
Object Definitions:
- Object is a real world entity.
- Object is a run time entity.
- Object is an entity which has state and behavior.
- Object is an instance of a class.
Class in Java
In Java everything is encapsulated under classes. Class is the core of Java language. Class can be defined as a template/ blueprint that describe the behaviors /states of a particular entity. A class defines new data type. Once defined this new type can be used to create object of that type. Object is an instance of class. You may also call it as physical existence of a logical template class.
A class is declared using class keyword. A class contain both data and code that operate on that data. The data or variables defined within a class are called instance variables and the code that operates on this data is known as methods. Thus, the instance variables and methods are known as class members. class is also known as a user defined datatype.
A class and an object can be related as follows: Consider an ice tray(like of cube shape) as a class. Then ice cubes can be considered as the objects which is a blueprint of its class i.e of ice tray.
Rules for Java Class
A class can have only public or default(no modifier) access specifier.
It can be either abstract, final or concrete (normal class).
It must have the class keyword, and class must be followed by a legal identifier.
It may optionally extend one parent class. By default, it will extend java.lang.Object.
It may optionally implement any number of comma-separated interfaces.
The class's variables and methods are declared within a set of curly braces {}.
Each .java source file may contain only one public class. A source file may contain any number of default visible classes.
Finally, the source file name must match the public class name and it must have a .java suffix.
A class in Java can contain:
- fields
- methods
- constructors
- blocks
- nested class and interface
Syntax:
class{ field; method; }
Instance variable in Java
Instance variable in java is used by Objects to store their states. Variables which are defined without the STATIC keyword and are Outside any method declaration are Object specific and are known as instance variables. They are called so because their values are instance specific and are not shared among instances.
class Page { public String pageName; // instance variable with public access private int pageNumber; // instance variable with private access } package com.jbt; /* * Here we will discuss about different type of Variables available in Java */ public class VariablesInJava { /* * Below variable is INSTANCE VARIABLE as it is outside any method and it is * not using STATIC modifier with it. It is using default access modifier. * To know more about ACCESS MODIFIER visit appropriate section */ int instanceField; /* * Below variable is STATIC variable as it is outside any method and it is * using STATIC modifier with it. It is using default access modifier. To * know more about ACCESS MODIFIER visit appropriate section */ static String staticField; public void method() { /* * Below variable is LOCAL VARIABLE as it is defined inside method in * class. Only modifier that can be applied on local variable is FINAL. * To know more about access and non access modifier visit appropriate * section. * * Note* : Local variable needs to initialize before they can be used. * Which is not true for Static or Instance variable. */ final String localVariable = "Initial Value"; System.out.println(localVariable); } public static void main(String args[]) { VariablesInJava obj = new VariablesInJava(); /* * Instance variable can only be accessed by Object of the class only as below. */ System.out.println(obj.instanceField); /* * Static field can be accessed in two way. * 1- Via Object of the class * 2- Via CLASS name */ System.out.println(obj.staticField); System.out.println(VariablesInJava.staticField); System.out.println(new VariablesInJava().instanceField); } }
Rules for Instance variable in Java
- Instance variables can use any of the four access levels
- They can be marked final
- They can be marked transient
- They cannot be marked abstract
- They cannot be marked synchronized
- They cannot be marked strictfp
- They cannot be marked native
- They cannot be marked static
Cheatsheet
Public, Private, Protected all 3 access modifiers can be applied to Instance Variable(Default also).
Instance variable will get default value means instance variable can be used without initializing it. Same is not true for Local Variable.
Instance Variable can be marked final.
Instance Variable can be marked transient.
Instance Variable cannot be abstract.
Instance Variable can not have synchronized modifier.
Instance Variable can not have strictfp modifier.
Instance Variable can not have the native modifier.
Instance Variable can not have Static modifier as it will become Class level variable.
Instance Variable Type | Default Value |
---|---|
boolean | false |
byte | (byte)0 |
short | (short) 0 |
int | 0 |
long | 0L |
char | u0000 |
float | 0.0f |
double | 0.0d |
Object | null |
Method in Java
In java, a method is like function i.e. used to expose behavior of an object.
Advantage of Method
- Code Reusability
- Code Optimization
new keyword in Java
The new keyword is used to allocate memory at run time. All objects get memory in Heap memory area.
Object and Class Example: main within class
In this example, we have created a Student class that have two data members id and name. We are creating the object of the Student class by new keyword and printing the objects value.
class Student{ int id;//field or data member or instance variable String name; public static void main(String args[]) { Student s1=new Student();//creating an object of Student System.out.println(s1.id);//accessing member through reference variable System.out.println(s1.name); } }
Output:
0 null
Object and Class Example: main outside class
In real time development, we create classes and use it from another class. It is a better approach than previous one. Let's see a simple example, where we are having main() method in another class.
We can have multiple classes in different java files or single java file. If you define multiple classes in a single java source file, it is a good idea to save the file name with the class name which has main() method.
class Student{ int id; String name; } class TestStudent { public static void main(String args[]){ Student s1=new Student(); System.out.println(s1.id); System.out.println(s1.name); } }
Output:
0 null
Ways to initialize object
There are 3 ways to initialize object in java.
- By reference variable
- By method
- By constructor
1)Object and Class Example: Initialization through reference
Initializing object simply means storing data into object. Let's see a simple example where we are going to initialize object through reference variable.
class Student{ int id; String name; } class TestStudent2{ public static void main(String args[]){ Student s1=new Student(); s1.id=31; s1.name="Rama"; System.out.println(s1.id+" "+s1.name);//printing members with a white space } }
Output:
31 Sonoo
We can also create multiple objects and store information in it through reference variable.
class Student{ int id; String name; } class TestStudent3{ public static void main(String args[]){ //Creating objects Student s1=new Student(); Student s2=new Student(); //Initializing objects s1.id=31; s1.name="Pavi"; s2.id=36; s2.name="Rama"; //Printing data System.out.println(s1.id+" "+s1.name); System.out.println(s2.id+" "+s2.name); } }
Output:
2) Object and Class Example: Initialization through method31 Pavi 36 Rama
In this example, we are creating the two objects of Student class and initializing the value to these objects by invoking the insertRecord method. Here, we are displaying the state (data) of the objects by invoking the displayInformation() method.
class Student{ int rollno; String name; void insertRecord(int r, String n){ rollno=r; name=n; } void displayInformation(){System.out.println(rollno+" "+name);} } class TestStudent4{ public static void main(String args[]){ Student s1=new Student(); Student s2=new Student(); s1.insertRecord(31,"Pavi"); s2.insertRecord(36,"Rama"); s1.displayInformation(); s2.displayInformation(); } }
Output:
31 Pavi 36 Rama
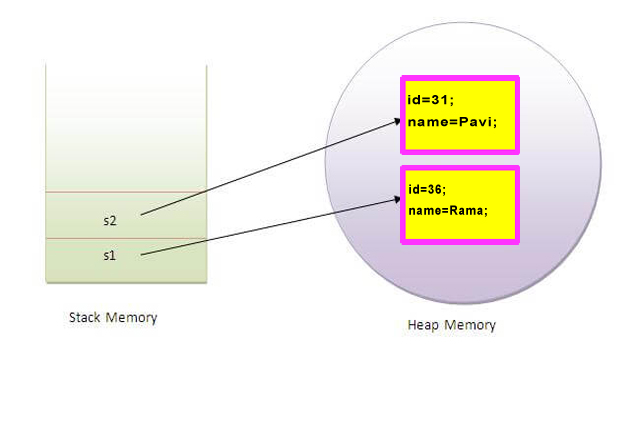
As you can see in the above figure, object gets the memory in heap memory area. The reference variable refers to the object allocated in the heap memory area. Here, s1 and s2 both are reference variables that refer to the objects allocated in memory.
3) Object and Class Example: Initialization through constructor
Constructors in Java
A constructor is a special method that is used to initialize an object.Every class has a constructor,if we don't explicitly declare a constructor for any java class the compiler builds a default constructor for that class. A constructor does not have any return type.
A constructor has same name as the class in which it resides. Constructor in Java can not be abstract, static, final or synchronized. These modifiers are not allowed for constructor.
class Car { String name ; String model; Car( ) //Constructor { name =""; model=""; } }
We will learn about constructors in java later.
Object and Class Example: Employee
class Employee{ int id; String name; float salary; void insert(int i, String n, float s) { id=i; name=n; salary=s; } void display(){System.out.println(id+" "+name+" "+salary);} } public class TestEmployee { public static void main(String[] args) { Employee e1=new Employee(); Employee e2=new Employee(); Employee e3=new Employee(); e1.insert(31,"Pavi",45000); e2.insert(36,"Rama",25000); e3.insert(3136,"PR",55000); e1.display(); e2.display(); e3.display(); } }
Output:
31 ajeet 45000.0 36 irfan 25000.0 3136 nakul 55000.0
Object and Class Example: Rectangle
class Rectangle{ int length; int width; void insert(int l, int w){ length=l; width=w; } void calculateArea(){System.out.println(length*width);} } class TestRectangle1{ public static void main(String args[]){ Rectangle r1=new Rectangle(); Rectangle r2=new Rectangle(); r1.insert(15,8); r2.insert(5,20); r1.calculateArea(); r2.calculateArea(); } }
Output:
120 100
What are the different ways to create an object in Java?
There are many ways to create an object in java. They are:
- By new keyword
- By newInstance() method
- By clone() method
- By deserialization
- By factory method etc.
We will learn these ways to create object later.
Anonymous object
Anonymous simply means nameless. An object which has no reference is known as anonymous object. It can be used at the time of object creation only.
If you have to use an object only once, anonymous object is a good approach. For example:
new Calculation();//anonymous object
Calling method through reference:
Calculation c=new Calculation(); c.fact(5);
Calling method through anonymous object
new Calculation().fact(5);
Let's see the full example of anonymous object in java.
class Calculation{ void fact(int n){ int fact=1; for(int i=1;i<=n;i++){ fact=fact*i; } System.out.println("factorial is "+fact); } public static void main(String args[]){ new Calculation().fact(10);//calling method with anonymous object } }
Output:
factorial is 3628800
Creating multiple objects by one type only
We can create multiple objects by one type only as we do in case of primitives.
Initialization of primitive variables:
int a=10, b=20;
Initialization of refernce variables:
Rectangle r1=new Rectangle(), r2=new Rectangle();//creating two objects
Example:
class Rectangle{ int length; int width; void insert(int l,int w){ length=l; width=w; } void calculateArea(){System.out.println(length*width);} } class TestRectangle10{ public static void main(String args[]){ Rectangle r1=new Rectangle(),r2=new Rectangle();//creating two objects r1.insert(11,5); r2.insert(3,15); r1.calculateArea(); r2.calculateArea(); } }
Output:
55 45
Real World Example: Account
class Account{ int acc_no; String name; float amount; void insert(int a,String n,float amt){ acc_no=a; name=n; amount=amt; } void deposit(float amt){ amount=amount+amt; System.out.println(amt+" deposited"); } void withdraw(float amt){ if(amount<amt){ System.out.println("Insufficient Balance"); }else{ amount=amount-amt; System.out.println(amt+" withdrawn"); } } void checkBalance(){System.out.println("Balance is: "+amount);} void display(){System.out.println(acc_no+" "+name+" "+amount);} } class TestAccount{ public static void main(String[] args){ Account a1=new Account(); a1.insert(733136,"Rama",5000); a1.display(); a1.checkBalance(); a1.deposit(80000); a1.checkBalance(); a1.withdraw(25000); a1.checkBalance(); } }
Output:
733136 Rama 5000.0 Balance is: 5000.0 80000.0 deposited Balance is: 85000.0 25000.0 withdrawn Balance is: 60000.0