A method is a collection of statements that perform some specific task and return result to the caller. A method can perform some specific task without returning anything. Methods allow us to reuse the code without retyping the code. In Java, every method must be part of some class which is different from languages like C, C++ and Python.
class Student { int roll; String name; Rank ranking; }
Method Declaration
Modifier-: Defines access type of the method i.e. from where it can be accessed in your application. In Java, there 4 type of the access specifiers.
- public: accessible in all class in your application.
- protected: accessible within the class in which it is defined and in its subclass(es)
- private: accessible only within the class in which it is defined.
- default (declared/defined without using any modifier) : accessible within same class and package within which its class is defined.
The return type : The data type of the value returned by the the method or void if does not return a value.
Method Name : the rules for field names apply to method names as well, but the convention is a little different.
Parameter list : Comma separated list of the input parameters are defined, preceded with their data type, within the enclosed parenthesis. If there are no parameters, you must use empty parentheses ().
Exception list : The exceptions you expect by the method can throw, you can specify these exception(s).
Method body : it is enclosed between braces. The code you need to be executed to perform your intended operations.
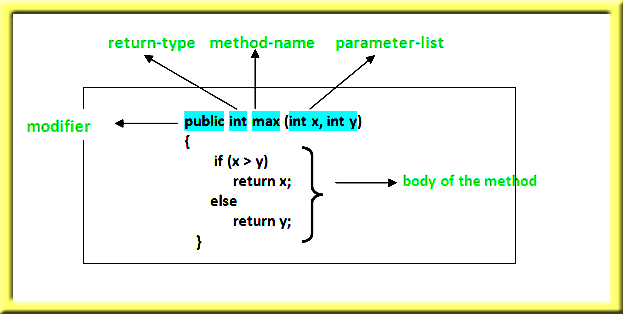
Method signature: It consists of method name and parameter list (number of parameters, type of the parameters and order of the parameters). Return type and exceptions are not considered as part of it.
Method Signature of above function:
max(int x, int y)
How to name a Method?: A method name is typically a single word that should be a verb in lowercase or multi-word, that begins with a verb in lowercase followed by adjective, noun….. After the first word, first letter of each word should be capitalized. For example, findSum,computeMax, setX and getX
Generally, A method has a unique name within the class in which it is defined but sometime a method might have same name as other method name within the same class as method overloading is allowed in Java.
Calling a method
The method needs to be called for using its functionality. There can be three situations when a method is called:
A method returns to the code that invoked it when:
- It completes all the statements in the method
- It reaches a return statement
- Throws an exception
// Program to illustrate methodsin java import java.io.*; class Addition { int sum = 0; public int addTwoInt(int a, int b) { sum = a + b; return sum; } } class GFG { public static void main (String[] args) { // creating an instance of Addition class Addition add = new Addition(); // calling addTwoInt() method to add two integer using instance created // in above step. int s = add.addTwoInt(5,3); System.out.println("Sum of two integer values :"+ s); } }
Output:
Sum of two integer values :8
// Java program to illustrate different ways of calling a method import java.io.*; class Test { public static int i = 0; // constructor of class which counts //the number of the objects of the class. Test() { i++; } public static int get() { return i; } public int m1() { System.out.println("Inside the method m1 by object of GFG class"); // calling m2() method within the same class. this.m2(); // statements to be executed if any return 1; } // It doesn't return anything as // return type is 'void'. public void m2() { System.out.println("In method m2 came from method m1"); } } class GFG { public static void main(String[] args) { // Creating an instance of the class Test obj = new Test(); // Calling the m1() method by the object created in above step. int i = obj.m1(); System.out.println("Control returned after method m1 :" + i); // Call m2() method // obj.m2(); int no_of_objects = Test.get(); System.out.print("No of instances created till now : "); System.out.println(no_of_objects); } }
Output:
Inside the method m1 by object of GFG class In method m2 came from method m1 Control returned after method m1 :1 No of instances created till now : 1
Memory allocation for methods calls
Methods calls are implemented through stack. Whenever a method is called a stack frame is created within the stack area and after that the arguments passed to and the local variables and value to be returned by this called method are stored in this stack frame and when execution of the called method is finished, the allocated stack frame would be deleted. There is a stack pointer register that tracks the top of the stack which is adjusted accordingly.
Java is Pass by Value and Not Pass by Reference
One of the biggest confusion in Java programming language is whether java is Pass by Value or Pass by Reference. I ask this question a lot in interviews and still see interviewee confused with it. So I thought to write a post about it to clarify all the confusions around it.
First of all we should understand what is meant by pass by value or pass by reference.
Pass by Value: The method parameter values are copied to another variable and then the copied object is passed, that’s why it’s called pass by value.
Pass by Reference: An alias or reference to the actual parameter is passed to the method, that’s why it’s called pass by reference.
package com.journaldev.test; public class Balloon { private String color; public Balloon(){} public Balloon(String c){ this.color=c; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } }
And we have a simple program with a generic method to swap two objects, the class looks like below.
package com.journaldev.test; public class Test { public static void main(String[] args) { Balloon red = new Balloon("Red"); //memory reference 50 Balloon blue = new Balloon("Blue"); //memory reference 100 swap(red, blue); System.out.println("red color="+red.getColor()); System.out.println("blue color="+blue.getColor()); foo(blue); System.out.println("blue color="+blue.getColor()); } private static void foo(Balloon balloon) { //baloon=100 balloon.setColor("Red"); //baloon=100 balloon = new Balloon("Green"); //baloon=200 balloon.setColor("Blue"); //baloon = 200 } //Generic swap method public static void swap(Object o1, Object o2){ Object temp = o1; o1=o2; o2=temp; } }
Output:
red color=Red blue color=Blue blue color=Red
If you look at the first two lines of the output, it’s clear that swap method didn’t worked. This is because Java is pass by value, this swap() method test can be used with any programming language to check whether it’s pass by value or pass by reference.
Let’s analyze the program execution step by step.
Balloon red = new Balloon("Red"); Balloon blue = new Balloon("Blue");
When we use new operator to create an instance of a class, the instance is created and the variable contains the reference location of the memory where object is saved. For our example, let’s assume that “red” is pointing to 50 and “blue” is pointing to 100 and these are the memory location of both Balloon objects.
Now when we are calling swap() method, two new variables o1 and o2 are created pointing to 50 and 100 respectively.
public static void swap(Object o1, Object o2){ //o1=50, o2=100 Object temp = o1; //temp=50, o1=50, o2=100 o1=o2; //temp=50, o1=100, o2=100 o2=temp; //temp=50, o1=100, o2=50 } //method terminated
Notice that we are changing values of o1 and o2 but they are copies of “red” and “blue” reference locations, so actually there is no change in the values of “red” and “blue” and hence the output.
If you have understood this far, you can easily understand the cause of confusion. Since the variables are just the reference to the objects, we get confused that we are passing the reference so java is pass by reference. However we are passing a copy of the reference and hence it’s pass by value. I hope it clear all the doubts now.
Now let’s analyze foo() method execution.
private static void foo(Balloon balloon) { //baloon=100 balloon.setColor("Red"); //baloon=100 balloon = new Balloon("Green"); //baloon=200 balloon.setColor("Blue"); //baloon = 200 }
The first line is the important one, when we call a method the method is called on the Object at the reference location. At this point, balloon is pointing to 100 and hence it’s color is changed to Red.
In the next line, ballon reference is changed to 200 and any further methods executed are happening on the object at memory location 200 and not having any effect on the object at memory location 100. This explains the third line of our program output printing blue color=Red.
I hope above explanation clear all the doubts, just remember that variables are references or pointers and it’s copy is passed to the methods, so java is always pass by value. It would be more clear when you will learn about Heap and Stack memory and where different objects and references are stored, for a detailed explanation with reference to a program, read Java Heap vs Stack.