Array Exercises
Write a C program to read elements in a matrix and check whether matrix is an Identity matrix or not. C program for finding Identity matrix. Logic to check identity matrix in C programming.
Required knowledge
Basic C programming, C for loop, ArrayS
What is Identity Matrix?
Identity matrix is a special square matrix whose main diagonal elements is equal to 1 and other elements are 0. Identity matrix is also known as unit matrix.
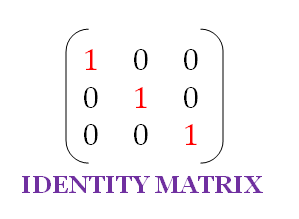
For checking a matrix A we need to ensure that if i = j then Aij must be equal to 1. Else Aij must be equal to 0. (Where 1 ≤ i ≤ m and 1 ≤ j ≤ n)
Program to check identity matrix
/** * C program to check whether a matrix is Identity matrix or not */ #include <stdio.h> #define SIZE 3 // Matrix size int main() { int A[SIZE][SIZE]; int row, col, isIdentity; /* Input elements in matrix from user */ printf("Enter elements in matrix of size 3x3: \n"); for(row=0; row<SIZE; row++) { for(col=0; col<SIZE; col++) { scanf("%d", &A[row][col]); } } /* Check whether it is Identity matrix or not */ isIdentity = 1; for(row=0; row<SIZE; row++) { for(col=0; col<SIZE; col++) { if(row==col && A[row][col]!=1) { /* If elements of main diagonal is not equal to 1 */ isIdentity = 0; } else if(row!=col && A[row][col]!=0) { /* If other elements than main diagonal is not equal to 0 */ isIdentity = 0; } } } /* If it is an Identity matrix */ if(isIdentity == 1) { printf("\nThe given matrix is an Identity Matrix.\n"); /* * Print the Identity matrix */ for(row=0; row<SIZE; row++) { for(col=0; col<SIZE; col++) { printf("%d ", A[row][col]); } printf("\n"); } } else { printf("The given matrix is not Identity Matrix"); } return 0; }
Output:
Enter elements in matrix of size 3x3:
1 0 0
0 1 0
0 0 1
The given matrix is an Identity Matrix.
1 0 0
0 1 0
0 0 1
Process returned 0 (0x0) execution time : 14.526 s
Enter elements in matrix of size 3x3:
1 0 1
0 1 0
0 0 1
The given matrix is not Identity Matrix
Process returned 0 (0x0) execution time : 15.919 s
Identity matrix is a special square matrix whose main diagonal elements is equal to 1 and other elements are 0. Identity matrix is also known as unit matrix.
Program to check identity matrix
/** * C program to check whether a matrix is Identity matrix or not */ #includeint main() { int A[10][10]; int row, col, isIdentity,n; printf("Enter size of matrix:"); scanf("%d",&n); /* Input elements in matrix from user */ printf("Enter elements in matrix: \n"); for(row=0; row<n; row++) { for(col=0; col<n; col++) { scanf("%d", &A[row][col]); } } /* Check whether it is Identity matrix or not */ isIdentity = 1; for(row=0; row<n; row++) { for(col=0; col<n; col++) { if(row==col && A[row][col]!=1) { /* If elements of main diagonal is not equal to 1 */ isIdentity = 0; } else if(row!=col && A[row][col]!=0) { /* If other elements than main diagonal is not equal to 0 */ isIdentity = 0; } } } /* If it is an Identity matrix */ if(isIdentity == 1) { printf("\nThe given matrix is an Identity Matrix.\n"); /* * Print the Identity matrix */ for(row=0; row<n; row++) { for(col=0; col<n; col++) { printf("%d ", A[row][col]); } printf("\n"); } } else { printf("The given matrix is not Identity Matrix"); } return 0; }